728x90
문제
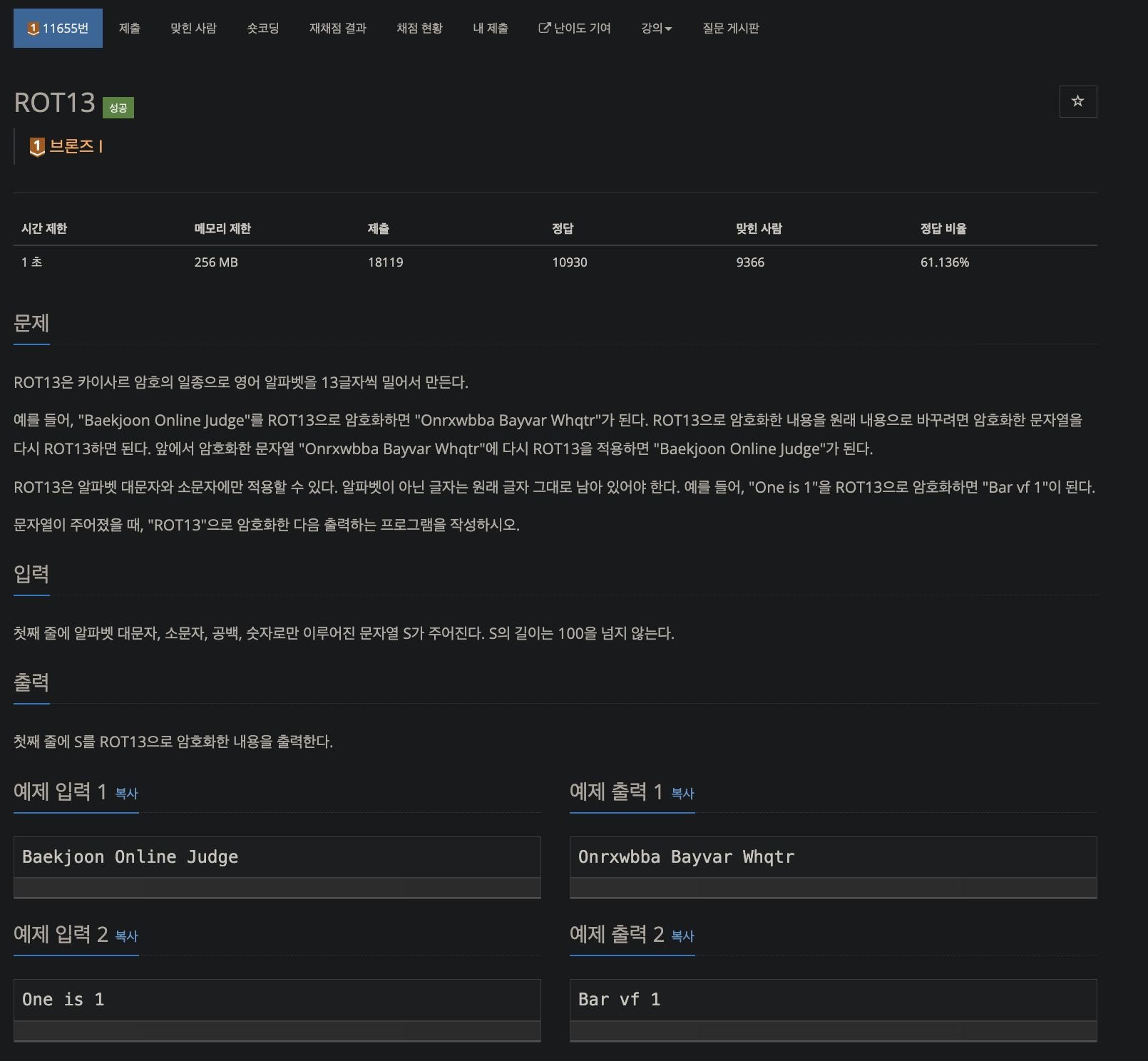
정답 코드
#include <iostream>
#include <string>
using namespace std;
int main()
{
cin.tie(0);
ios:: sync_with_stdio(0);
string s;
getline(cin, s);
for (int i=0; i < s.size(); i++)
{
if ('a'<= s[i] && s[i] <= 'a' + 12)
s[i] +=13;
else if ('a' + 13 <= s[i] && s[i] <= 'z')
s[i] -=13;
else if ('A'<= s[i] && s[i] <= 'A' + 12)
s[i] +=13;
else if ('A' + 13<= s[i] && s[i] <= 'Z')
s[i] -=13;
}
cout << s;
}
문제 풀이의 흐름
흐름 자체는 간단한 문제이다.
- 문자열을 개행까지 쭉 받아준다.
- 알파벳은 26개이므로 대문자의 a부터 a+ 12까지는 13을 더해주고 그 뒤는 13을 빼준다.
- 바꾼 문자열을 출력한다.
주의 할 점
cin 은 공백 전까지 입력을 받는다.
이걸 몰라서 처음에 꽤나 당황을 했었다.
처음으로 getline함수를 사용해봤고 사용방법은 제가 정리한 string 함수 정리에 잘 적혀있다.(링크)
이 부분만 주의 하고 아스키코드값을 넘어갈 때 빼주는 것을 잘 생각했다면 문제는 안 일어날 것 같다.
반성 및 고찰
getline함수에 대해서 모르고 있었다. 이번 기회에 정리를 해보아서 도움이 됐다.
추가적으로 이터레이터에 직접 값을 더해줬는데 char형 복사한 값에 더해주는 말이 안되는 코드였다.
실패한 코드
#include <iostream>
#include <string>
using namespace std;
int main()
{
cin.tie(0);
ios:: sync_with_stdio(0);
string s;
getline(cin, s);
for (auto it : s)
{
if ('a'<= it && it <= 'a' + 12)
it +=13;
else if ('a' + 13<= it && it <= 'z')
it -=13;
else if ('A'<= it && it <= 'A' + 12)
it +=13;
else if ('A' + 13<= it && it <= 'Z')
it -=13;
}
cout << s;
}
하지만 이터레이터를 이용해서 하는게 반드시 실패하는건 아니다.
바로 레퍼런스 타입을 주게 된다면 성공하는 코드로 바꿀 수 있다.
수정 후 성공
#include <iostream>
#include <string>
using namespace std;
int main()
{
cin.tie(0);
ios:: sync_with_stdio(0);
string s;
getline(cin, s);
for (auto &it : s)
{
if ('a'<= it && it <= 'a' + 12)
it +=13;
else if ('a' + 13<= it && it <= 'z')
it -=13;
else if ('A'<= it && it <= 'A' + 12)
it +=13;
else if ('A' + 13<= it && it <= 'Z')
it -=13;
}
cout << s;
}
야호!
Uploaded by N2T
728x90
'알고리즘 기초시절' 카테고리의 다른 글
백준 8958(C) strlen함수사용 (0) | 2023.01.03 |
---|---|
백준 A+B-4 문제 10951번 EOF (0) | 2023.01.03 |
백준 1159번 농구경기 (C++) (0) | 2022.12.30 |
백준 10988번 팰린드롬인지 확인하기(C++) (0) | 2022.12.29 |
백준 2979번 트럭주차 (C++) (0) | 2022.12.29 |